Maximum subarray problem
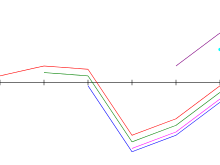
In computer science, the maximum subarray problem is the task of finding the contiguous subarray within a one-dimensional array, a[1...n], of numbers which has the largest sum. Formally, the task is to find with , such that the sum
is maximal.
The array usually contains both positive and negative numbers along with 0. For example, for the array of values −2, 1, −3, 4, −1, 2, 1, −5, 4; the contiguous subarray with the largest sum is 4, −1, 2, 1, with sum 6.
Some properties of this problem are:
- If the array contains all non-positive numbers, then the solution is the number in the array with the smallest magnitude.
- If the array contains all non-negative numbers, then the problem is trivial and the maximum sum is the sum of all the elements in the list.
- An empty set is not valid.
- There can be multiple different sub-arrays that achieve the same maximum sum to the problem.
There will be discussions on different algorithm techniques that are used to solve this problem which include: brute force algorithm, divide and conquer, and dynamic programming. This analysis will compare the time complexity of the different algorithms for the MSP.
History
The problem was first posed by Ulf Grenander of Brown University in 1977 as a simplified model for maximum likelihood estimation of patterns in digitized images. Grenander was looking to find the maximum sum of an m * n rectangular region of real numbers. He designed an algorithm that ran in O(n6) time. His algorithm was too slow and the problem was too complex to be solved, so he simplified it to one dimension. This made the problem easier to comprehend and as a result, he was able to solve the original problem with a faster running time of O(n3). Jay Kadane of Carnegie Mellon University (Bentley 1984) soon after designed a linear time algorithm.
Algorithms to solve a two dimensional version of the problem were later designed and run in O(n3) time, using Kadane's algorithm or a divide and conquer approach. Overall the optimal running time for a one dimensional array is O(n), while the two dimensional array has been improved to a sub-cubic time by the efforts of Tamaki and Tokuyama.[1]
Using the Bird–Meertens formalism, an O(n) algorithm has been derived by algebraic manipulation of the brute-force algorithm in a functional programming language.[2]
Applications
Maximum subarray algorithms are used for data analysis in many fields, such as genomic sequence analysis, computer vision, and data mining.
Genomic sequence analysis employs maximum subarray algorithms to identify important biological segments of protein sequences and the information for the purpose of predicting outcomes. As an example, specific information of a protein sequence can be organized into a linear function which can be used to understand the structure and function of a protein. Biologists find this approach to be efficient and easy to analyze their data.
The score-based technique of finding the segment with the highest total sum is used in many problems similar to the MSP. In genomic sequence analysis, these problems include conserved segments, GC-rich regions, tandem repeats, low-complexity filter, DNA binding domains, and regions of high charge.
For computer vision , maximum subarray algorithms are used in bitmap images to detect the highest score subsequence which represents the brightest area in an image. The image is a two dimensional array of positive values that corresponds to the brightness of a pixel. The algorithm is evaluated after normalizing every value in the array by subtracting them from the mean brightness.
Data mining is an application of maximum subarray algorithms with numerical attributes. To understand the role of the maximum subarray problem in data mining it is important to be familiar with the association rule and its parts. The association rule is an if/then statement that creates relationships between unrelated pieces of data. The two parts of the association rule are the antecedent (if statement) and the consequent (then statement). In business applications of data mining, association rules are important in examining and foreseeing customer's actions/behavior. Data mining is used for companies to anticipate common trends, by representing problems with maximum subarray problem into an sub-array to be normalized and solved. The highest result of the array will give companies information of what customers are responding well to and will influence the companies' actions as a result.
Kadane's algorithm
A bit of a background: Kadane's algorithm is based on splitting up the set of possible solutions into mutually exclusive (disjoint) sets. It exploits the fact that any solution (i.e., any member of the set of solutions) will always have a last element (this is what is meant by "sum ending at position "). Thus, we simply have to examine, one by one, the set of solutions whose last element's index is , the set of solutions whose last element's index is , then , and so forth to . It turns out that this process can be carried out in linear time.
Kadane's algorithm begins with a simple inductive question: if we know the maximum subarray sum ending at position (call this ), what is the maximum subarray sum ending at position (equivalently, what is )? The answer turns out to be relatively straightforward: either the maximum subarray sum ending at position includes the maximum subarray sum ending at position as a prefix, or it doesn't (equivalently, , where is the element at index ).
Thus, we can compute the maximum subarray sum ending at position for all positions by iterating once over the array. As we go, we simply keep track of the maximum sum we've ever seen. Thus, the problem can be solved with the following code, expressed here in Python:
1 def max_subarray(A):
2 max_ending_here = max_so_far = A[0]
3 for x in A[1:]:
4 max_ending_here = max(x, max_ending_here + x)
5 max_so_far = max(max_so_far, max_ending_here)
6 return max_so_far
Note: with a bit of reasoning you will see that max_so_far
is equal to
.
The algorithm can also be easily modified to keep track of the starting and ending indices of the maximum subarray (when max_so_far
changes) as well as the case where we want to allow zero-length subarrays (with implicit sum 0) if all elements are negative.
Because of the way this algorithm uses optimal substructures (the maximum subarray ending at each position is calculated in a simple way from a related but smaller and overlapping subproblem: the maximum subarray ending at the previous position) this algorithm can be viewed as a simple/trivial example of dynamic programming.
The runtime complexity of Kadane's algorithm is [3].
Generalizations
Similar problems may be posed for higher-dimensional arrays, but their solutions are more complicated; see, e.g., Takaoka (2002). Brodal & Jørgensen (2007) showed how to find the k largest subarray sums in a one-dimensional array, in the optimal time bound .
The Maximum sum k-disjoint subarrays can also be computed in the optimal time bound .[4]
See also
References
- ↑ Tamaki, H.; Tokuyama, T. (1998). "Algorithms for the Maximum Subarray Problem Based on Matrix Multiplication". Proceedings of the 9th SODA (Symposium on Discrete Algorithms): 446–452.
|access-date=
requires|url=
(help) - ↑ Richard S. Bird (1989). "Algebraic Identities for Program Calculation" (PDF). The Computer Journal. 32 (2): 122&mdash, 126. Here: Sect.8, p.126
- ↑ https://www.khanacademy.org/computing/computer-science/algorithms/asymptotic-notation/a/big-o-notation
- ↑ Bengtsson, Fredrik; Chen, Jingsen (2007). "Computing maximum-scoring segments optimally" (PDF). Luleå University of Technology (3).
- Bentley, Jon (1984), "Programming pearls: algorithm design techniques", Communications of the ACM, 27 (9): 865–873, doi:10.1145/358234.381162 .
- Brodal, Gerth Stølting; Jørgensen, Allan Grønlund (2007), "A linear time algorithm for the k maximal sums problem", Mathematical Foundations of Computer Science 2007, Lecture Notes in Computer Science, 4708, Springer-Verlag, pp. 442–453, doi:10.1007/978-3-540-74456-6_40 .
- Takaoka, T. (2002), "Efficient algorithms for the maximum subarray problem by distance matrix multiplication" (PDF), Electronic Notes in Theoretical Computer Science, 61 .
- TAN, Lirong. "Maximum Contiguous Subarray Sum Problems" (PDF).
- Mu, Shin-Cheng (2010). "The Maximum Segment Sum Problem: Its Origin, and a Derivation".
- Bae, Sung Eun (2007). "Sequential and Parallel Algorithms for the Generalized Maximum Subarray Problem" (PDF).
- "Notes on Maximum Subarray Problem". 2012.