Builder pattern
The Builder is a design pattern designed to provide a flexible solution to various object creation problems in object-oriented programming. The intent of the Builder design pattern is to separate the construction of a complex object from its representation. It is one of the Gang of Four design patterns.
Overview
The Builder design pattern is one of the twenty-three well-known GoF design patterns[1] that describe how to solve recurring design problems in object-oriented software.
The Builder design pattern solves problems like:[2]
- How can a class (the same construction process) create different representations of a complex object?
- How can a class that includes creating a complex object be simplified?
Creating and assembling the parts of a complex object directly within a class is inflexible. It commits the class to creating a particular representation of the complex object and makes it impossible to change the representation later independently from (without having to change) the class.
The Builder design pattern describes how to solve such problems:
- Encapsulate creating and assembling the parts of a complex object in a separate
Builder
object. - A class delegates object creation to a
Builder
object instead of creating the objects directly.
A class (the same construction process) can delegate to different Builder
objects to create different representations of a complex object.
Definition
The intent of the Builder design pattern is to separate the construction of a complex object from its representation. By doing so the same construction process can create different representations.[1]
Advantages
Advantages of the Builder pattern include:[3]
- Allows you to vary a product’s internal representation.
- Encapsulates code for construction and representation.
- Provides control over steps of construction process.
Disadvantages
Disadvantages of the Builder pattern include:[3]
- Requires creating a separate ConcreteBuilder for each different type of product.
- Requires the builder classes to be mutable.
- Data members of class aren't guaranteed to be initialized.
- Dependency injection may be less supported.
Structure
UML class and sequence diagram
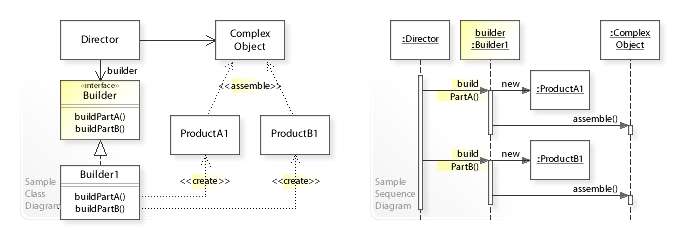
In the above UML class diagram,
the Director
class doesn't create and assemble the ProductA1
and ProductB1
objects directly.
Instead, the Director
refers to the Builder
interface for building (creating and assembling) the parts of a complex object,
which makes the Director
independent of which concrete classes are instantiated (which representation is created).
The Builder1
class implements the Builder
interface by creating and assembling the ProductA1
and ProductB1
objects.
The UML sequence diagram shows the run-time interactions:
The Director
object calls buildPartA()
on the Builder1
object, which creates and assembles the ProductA1
object.
Thereafter,
the Director
calls buildPartB()
on Builder1
, which creates and assembles the ProductB1
object.
Class diagram
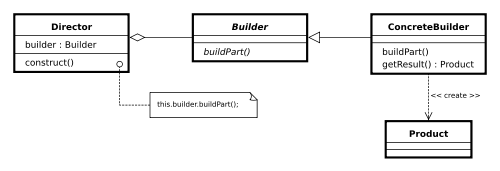
- Builder
- Abstract interface for creating objects (product).
- ConcreteBuilder
- Provides implementation for Builder. It is an object able to construct other objects. Constructs and assembles parts to build the objects.
Pseudocode
We have a Car class. The problem is that a car has many options. The combination of each option would lead to a huge list of constructors for this class. So we will create a builder class, CarBuilder. We will send to the CarBuilder each car option step by step and then construct the final car with the right options:
class Car is
Can have GPS, trip computer and various numbers of seats.
Can be a city car, a sports car, or a cabriolet.
class CarBuilder is
method getResult() is
output: a Car with the right options
Construct and return the car.
method setSeats(number) is
input: the number of seats the car may have.
Tell the builder the number of seats.
method setCityCar() is
Make the builder remember that the car is a city car.
method setCabriolet() is
Make the builder remember that the car is a cabriolet.
method setSportsCar() is
Make the builder remember that the car is a sports car.
method setTripComputer() is
Make the builder remember that the car has a trip computer.
method unsetTripComputer() is
Make the builder remember that the car does not have a trip computer.
method setGPS() is
Make the builder remember that the car has a global positioning system.
method unsetGPS() is
Make the builder remember that the car does not have a global positioning system.
Construct a CarBuilder called carBuilder
carBuilder.setSeats(2)
carBuilder.setSportsCar()
carBuilder.setTripComputer()
carBuilder.unsetGPS()
car := carBuilder.getResult()
So this indicates that the Builder pattern is more than just a means to limit constructor proliferation. It removes what could be a complex building process from being the responsibility of the user of the object that is built. It also allows for inserting new implementations of how an object is built without disturbing the client code.
Examples
C#
/// <summary>
/// Represents a product created by the builder
/// </summary>
public class Car
{
public string Make { get; set; }
public string Model { get; set; }
public int NumDoors { get; set; }
public string Colour { get; set; }
public Car(string make, string model, string colour, int numDoors)
{
Make = make;
Model = model;
Colour = colour;
NumDoors = numDoors;
}
}
/// <summary>
/// The builder abstraction
/// </summary>
public interface ICarBuilder
{
string Colour { get; set; }
int NumDoors { get; set; }
Car GetResult();
}
/// <summary>
/// Concrete builder implementation
/// </summary>
public class FerrariBuilder : ICarBuilder
{
public string Colour { get; set; }
public int NumDoors { get; set; }
public Car GetResult()
{
return NumDoors == 2 ? new Car("Ferrari", "488 Spider", Colour, NumDoors) : null;
}
}
/// <summary>
/// The director
/// </summary>
public class SportsCarBuildDirector
{
private ICarBuilder _builder;
public SportsCarBuildDirector(ICarBuilder builder)
{
_builder = builder;
}
public void Construct()
{
_builder.Colour = "Red";
_builder.NumDoors = 2;
}
}
public class Client
{
public void DoSomethingWithCars()
{
var builder = new FerrariBuilder();
var director = new SportsCarBuildDirector(builder);
director.Construct();
Car myRaceCar = builder.GetResult();
}
}
The Director assembles a car instance in the example above, delegating the construction to a separate builder object that it has been given to the Director by the Client.
C++
////// Product declarations and inline impl. (possibly Product.h) //////
class Product{
public:
// use this class to construct Product.
class Builder;
private:
// variables in need of initialization to make valid object
const int i;
const float f;
const char c;
// Only one simple constructor - rest is handled by Builder
Product( const int i, const float f, const char c ) : i(i), f(f), c(c){}
public:
// Product specific functionality
void print();
void doSomething();
void doSomethingElse();
};
class Product::Builder{
private:
// variables needed for construction of object of Product class
int i;
float f;
char c;
// default values for variables
static constexpr int defaultI = 1;
static constexpr float defaultF = 3.1415f;
static constexpr char defaultC = 'a';
public:
// create Builder with default values assigned
// (in C++11 they can be simply assigned above on declaration instead)
Builder() : i( defaultI ), f( defaultF ), c( defaultC ){ }
// sets custom values for Product creation
// returns Builder for shorthand inline usage (same way as cout <<)
Builder& setI( int i ){ this->i = i; return *this; }
Builder& setF( float f ){ this->f = f; return *this; }
Builder& setC( char c ){ this->c = c; return *this; }
// prepare specific frequently desired Product
// returns Builder for shorthand inline usage (same way as cout <<)
Builder& setProductP(){
i = 42;
f = -1.0f/12.0f;
c = '@';
return *this;
}
// produce desired Product
Product build(){
// Here, optionaly check variable consistency
// and also if Product is buildable from given information
return Product( i, f, c );
}
};
///// Product implementation (possibly Product.cpp) /////
#include <iostream>
void Product::print(){
using namespace std;
cout << "Product internals dump:" << endl;
cout << "i: " << i << endl;
cout << "f: " << f << endl;
cout << "c: " << c << endl;
}
void Product::doSomething(){}
void Product::doSomethingElse(){}
//////////////////// Usage of Builder (replaces Director from diagram)
int main(){
// simple usage
Product p1 = Product::Builder().setI(2).setF(0.5f).setC('x').build();
p1.print(); // test p1
// advanced usage
Product::Builder b;
b.setProductP();
Product p2 = b.build(); // get Product P object
b.setC('!'); // customize Product P
Product p3 = b.build();
p2.print(); // test p2
p3.print(); // test p3
}
Crystal
class Car
property wheels : Int32
property seats : Int32
property color : String
def initialize(@wheels = 4, @seats = 4, @color = "Black")
end
end
abstract class Builder
abstract def set_wheels(number : Int32)
abstract def set_seats(number : Int32)
abstract def set_color(color : String)
abstract def get_result
end
class CarBuilder < Builder
private getter car : Car
def initialize
@car = Car.new
end
def set_wheels(value : Int32)
@car.wheels = value
end
def set_seats(value : Int32)
@car.seats = value
end
def set_color(value : String)
@car.color = value
end
def get_result
return @car
end
end
class CarBuilderDirector
def self.construct : Car
builder = CarBuilder.new
builder.set_wheels(8)
builder.set_seats(4)
builder.set_color("Red")
builder.get_result
end
end
car = CarBuilderDirector.construct
p car
F#
1 /// <summary>
2 /// Represents a product created by the builder
3 /// </summary>
4 type Car () =
5 member val Wheels = 0 with get,set
6 member val Colour = null with get,set
7
8
9 // F# can actually enforce non-null strings being passed
10 //this doesn't show the full blown pattern, it just makes passing a null string a difficult/obvious syntax
11 module SpecialStrings =
12 type NonNullString = |NonNullString of string
13 // shadow the constructor
14 let NonNullString x =
15 match x with
16 | null -> None
17 | x -> Some (NonNullString x)
18
19 /// <summary>
20 /// The builder abstraction
21 /// </summary>
22 type ICarBuilder =
23 abstract member SetColour: SpecialStrings.NonNullString -> unit
24 abstract member SetWheels: int -> unit
25 // return an option type, in case current values aren't valid to create a Car
26 abstract member GetResult: unit -> Car option
27
28 /// <summary>
29 /// Concrete builder implementation
30 /// </summary>
31 type CarBuilder () =
32 let car = Car()
33 member x.SetColour (NonNullString colour) =
34 car.Colour <- colour
35 member x.SetWheels count =
36 car.Wheels <- count
37 member x.GetResult() = if not <| isNull car.Colour then Some car else None
38
39 interface ICarBuilder with
40 member x.SetColour = x.SetColour
41 member x.SetWheels = x.SetWheels
42 member x.GetResult = x.GetResult
43
44 /// <summary>
45 /// The director
46 /// </summary>
47 type CarBuildDirector () =
48 member x.Construct() =
49 let builder = CarBuilder()
50 NonNullString "Red"
51 |> Option.get
52 |> builder.SetColour
53 builder.SetWheels 4
54 builder.GetResult()
In F# we can enforce that a function or method cannot be called with specific data.[5] The example shows a simple way to do it that doesn't fully block a caller from going around it. This way just makes it obvious when someone is doing so in a code base.
Java
/**
* Represents the product created by the builder.
*/
class Car {
private int wheels;
private String color;
public Car() {
}
public String getColor() {
return color;
}
public void setColor(final String color) {
this.color = color;
}
public int getWheels() {
return wheels;
}
public void setWheels(final int wheels) {
this.wheels = wheels;
}
@Override
public String toString() {
return "Car [wheels = " + wheels + ", color = " + color + "]";
}
}
/**
* The builder abstraction.
*/
interface CarBuilder {
Car build();
CarBuilder setColor(final String color);
CarBuilder setWheels(final int wheels);
}
class CarBuilderImpl implements CarBuilder {
private Car car;
public CarBuilderImpl() {
car = new Car();
}
@Override
public Car build() {
return car;
}
@Override
public CarBuilder setColor(final String color) {
car.setColor(color);
return this;
}
@Override
public CarBuilder setWheels(final int wheels) {
car.setWheels(wheels);
return this;
}
}
public class CarBuildDirector {
private CarBuilder builder;
public CarBuildDirector(final CarBuilder builder) {
this.builder = builder;
}
public Car construct() {
return builder.setWheels(4)
.setColor("Red")
.build();
}
public static void main(final String[] arguments) {
final CarBuilder builder = new CarBuilderImpl();
final CarBuildDirector carBuildDirector = new CarBuildDirector(builder);
System.out.println(carBuildDirector.construct());
}
}
Scala
/**
* Represents the product created by the builder.
*/
case class Car(wheels:Int, color:String)
/**
* The builder abstraction.
*/
trait CarBuilder {
def setWheels(wheels:Int) : CarBuilder
def setColor(color:String) : CarBuilder
def build() : Car
}
class CarBuilderImpl extends CarBuilder {
private var wheels:Int = 0
private var color:String = ""
override def setWheels(wheels:Int) = {
this.wheels = wheels
this
}
override def setColor(color:String) = {
this.color = color
this
}
override def build = Car(wheels,color)
}
class CarBuildDirector(private val builder : CarBuilder) {
def construct = builder.setWheels(4).setColor("Red").build
def main(args: Array[String]): Unit = {
val builder = new CarBuilderImpl
val carBuildDirector = new CarBuildDirector(builder)
println(carBuildDirector.construct)
}
}
Python
from __future__ import print_function
from abc import ABCMeta, abstractmethod
class Car(object):
def __init__(self, wheels=4, seats=4, color="Black"):
self.wheels = wheels
self.seats = seats
self.color = color
def __str__(self):
return "This is a {0} car with {1} wheels and {2} seats.".format(
self.color, self.wheels, self.seats
)
class Builder:
__metaclass__ = ABCMeta
@abstractmethod
def set_wheels(self, value):
pass
@abstractmethod
def set_seats(self, value):
pass
@abstractmethod
def set_color(self, value):
pass
@abstractmethod
def get_result(self):
pass
class CarBuilder(Builder):
def __init__(self):
self.car = Car()
def set_wheels(self, value):
self.car.wheels = value
return self
def set_seats(self, value):
self.car.seats = value
return self
def set_color(self, value):
self.car.color = value
return self
def get_result(self):
return self.car
class CarBuilderDirector(object):
@staticmethod
def construct():
return CarBuilder()
.set_wheels(8)
.set_seats(4)
.set_color("Red")
.get_result()
car = CarBuilderDirector.construct()
print(car)
See also
References
- 1 2 Erich Gamma, Richard Helm, Ralph Johnson, John Vlissides (1994). Design Patterns: Elements of Reusable Object-Oriented Software. Addison Wesley. pp. 97ff. ISBN 0-201-63361-2.
- ↑ "The Builder design pattern - Problem, Solution, and Applicability". w3sDesign.com. Retrieved 2017-08-13.
- 1 2 "Index of /archive/2010/winter/51023-1/presentations" (PDF). www.classes.cs.uchicago.edu. Retrieved 2016-03-03.
- ↑ "The Builder design pattern - Structure and Collaboration". w3sDesign.com. Retrieved 2017-08-12.
- ↑ "Designing with types: Single case union types | F# for fun and profit". fsharpforfunandprofit.com. Retrieved 2017-03-30.
External links
![]() |
The Wikibook Computer Science Design Patterns has a page on the topic of: Builder implementations in various languages |
- Builder pattern implementation in Java
- The JavaWorld article Build user interfaces without getters and setters (Allen Holub) shows the complete Java source code for a Builder.
- Item 2: Consider a builder by Joshua Bloch