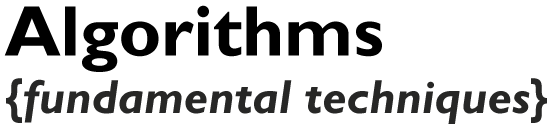
Preamble
This book aims to be an accessible introduction into the design and analysis of efficient algorithms. Throughout the book we will introduce only the most basic techniques and describe the rigorous mathematical methods needed to analyze them.
The topics covered include:
- The divide and conquer technique.
- The use of randomization in algorithms.
- The general, but typically inefficient, backtracking technique.
- Dynamic programming as an efficient optimization for some backtracking algorithms.
- Greedy algorithms as an optimization of other kinds of backtracking algorithms.
- Hill-climbing techniques, including network flow.
The goal of the book is to show you how you can methodically apply different techniques to your own algorithms to make them more efficient. While this book mostly highlights general techniques, some well-known algorithms are also looked at in depth. This book is written so it can be read from "cover to cover" in the length of a semester, where sections marked with a *
may be skipped.
This book is a tutorial on techniques and is not a reference. For references we highly recommend the tomes by [Knuth] and [CLRS]. Additionally, sometimes the best insights come from the primary sources themselves (e.g. [Hoare]).
Table of Chapters
Why a Wikibook on Algorithms?
A wikibook is an undertaking similar to an open-source software project: A contributor creates content for the project to help others, for personal enrichment, or to accomplish something for the contributor's own work (e.g., lecture preparation).
An open book, just like an open program, requires time to complete, but it can benefit greatly from even modest contributions from readers. For example you can fix "bugs" in the text (where the bug might be typographic, expository, technical, aesthetic or otherwise) in order to make a better book. If you find an opportunity to fix a bug, simply click on "edit", make your changes, and click on save. Other contributors may review your changes to be sure they are appropriate for the book. If you are unsure, you can visit the discussion page and ask there. Use common sense.
If you would like to make bigger contributions, you can take a look at the sections or chapters that are too short or otherwise need more work and start writing! Be sure to skim the rest of the book first in order to avoid duplication of content. Additionally, you should read the Guidelines for Contributors page for consistency tips and advice.
Note that you don't need to contribute everything at once. You can mark sections as "TODO," with a description of what remains to be done, and perhaps someone else will finish those parts for you. Once all TODO items are finished, we'll have reached our First Edition!
This book is intentionally kept narrow-in-focus in order to make contributions easier (because then the end-goal is clearer). This book is part two of a series of three computer science textbooks on algorithms, starting with Data Structures and ending with Advanced Data Structures and Algorithms. If you would like to contribute a topic not already listed in any of the three books try putting it in the Advanced book, which is more eclectic in nature. Or, if you think the topic is fundamental, you can go to either the Algorithms discussion page or the Data Structures discussion page and make a proposal.
Additionally, implementations of the algorithms as an appendix are welcome.
Further reading
The following books list Algorithms as a prerequisite:
Sources
The following sources are used with permission from the original authors. Some of the sources have been edited (sometimes heavily) from the initial versions, and thus all mistakes are our own.
[Impagliazzo] | Russell Impagliazzo. Lecture notes from algorithms courses 101 (Spring 2004; undergraduate), and 202 (Spring 2004, Fall 2004; graduate). University of California, San Diego. Used almost everywhere. |
[Lippert] | Eric Lippert. "Recursion and Dynamic Programming," from Fabulous Adventures In Coding. 21 July 2004. Used with permission. http://blogs.msdn.com/b/ericlippert/archive/2004/07/21/recursion-and-dynamic-programming.aspx Used in the backtracking and dynamic programming chapters. |
[Wikipedia] | Wikipedia, the free encyclopedia. http://www.wikipedia.org. Quoted/appropriated pervasively. |
References
The authors highly recommend the following reference materials.
[Aho] | Alfred V. Aho, Jeffrey D. Ullman, John E. Hopcroft. Data Structures and Algorithms. Addison Wesley, 1983. |
[CLRS] | Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, Clifford Stein. Introduction to Algorithms. McGraw-Hill, 2001. |
[Hoare] | C. A. R. Hoare. "Algorithm 63: Partition", "Algorithm 64: Quicksort", "Algorithm 65: Find", from Communications of the ACM. Volume 4, Issue 7 (July 1961). Page 321. ISSN:0001-0782. http://doi.acm.org/10.1145/366622.366642. |
[Knuth] | Donald E. Knuth. The Art of Computer Programming, Volumes 1-3. Addison-Wesley Professional, 1998. |
Contributors
Macneil Shonle A large portion of my contributions here come from lectures made by [Impagliazzo] at UCSD. I like this project because it gives me a chance to explain algorithms in the way that I finally understood them
Matthew Wilson I typed in an outline after finishing a graduate algorithms course. M. Shonle has taken this textbook and really made it something great.
Martin Krischik I supplied the Ada examples for the algorithms. You never know if an algorithm works until you have actually implemented it.
See also the Sources list for contributions used with permission.
External links
- Algorithms by S. Dasgupta, C.H. Papadimitriou, and U.V. Vazirani
- Introduction to Algorithms by C.E. Leiserson