Lorenz 96 model
The Lorenz 96 model is a dynamical system formulated by Edward Lorenz in 1996.[1] It is defined as follows. For :
where it is assumed that and . Here is the state of the system and is a forcing constant. is a common value known to cause chaotic behavior.
It is commonly used as a model problem in data assimilation.[2]
Python simulation
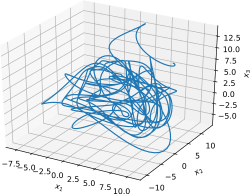
Plot of the first three variables of the simulation
from mpl_toolkits.mplot3d import Axes3D
from scipy.integrate import odeint
import matplotlib.pyplot as plt
import numpy as np
# These are our constants
N = 36 # Number of variables
F = 8 # Forcing
def lorenz96(x, t):
"""Lorenz 96 model."""
# Compute state derivatives
d = np.zeros(N)
# First the 3 edge cases: i=1,2,N
d[0] = (x[1] - x[N-2]) * x[N-1] - x[0]
d[1] = (x[2] - x[N-1]) * x[0] - x[1]
d[N-1] = (x[0] - x[N-3]) * x[N-2] - x[N-1]
# Then the general case
for i in range(2, N-1):
d[i] = (x[i+1] - x[i-2]) * x[i-1] - x[i]
# Add the forcing term
d = d + F
# Return the state derivatives
return d
x0 = F * np.ones(N) # Initial state (equilibrium)
x0[19] += 0.01 # Add small perturbation to 20th variable
t = np.arange(0.0, 30.0, 0.01)
x = odeint(lorenz96, x0, t)
# Plot the first three variables
fig = plt.figure()
ax = fig.gca(projection='3d')
ax.plot(x[:, 0], x[:, 1], x[:, 2])
ax.set_xlabel('$x_1$')
ax.set_ylabel('$x_2$')
ax.set_zlabel('$x_3$')
plt.show()
References
- Lorenz, Edward (1996). "Predictability – A problem partly solved" (PDF). Seminar on Predictability, Vol. I, ECMWF.
- Ott, Edward; et al. "A Local Ensemble Kalman Filter for Atmospheric Data Assimilation". arXiv:physics/0203058.
This article is issued from Wikipedia. The text is licensed under Creative Commons - Attribution - Sharealike. Additional terms may apply for the media files.