< Ada Programming
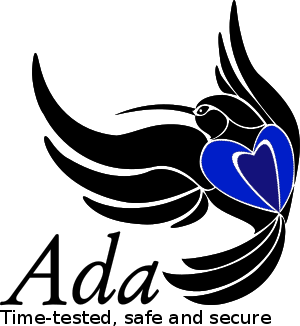
Ada. Time-tested, safe and secure.
function
Generate_Number (MaxValue : Integer)return
Integeris
subtype
Random_Typeis
Integerrange
0 .. MaxValue;package
Random_Packis
new
Ada.Numerics.Discrete_Random (Random_Type); G : Random_Pack.Generator;begin
Random_Pack.Reset (G);return
Random_Pack.Random (G);end
Generate_Number;function
Generate_Number (MinValue : Integer; MaxValue : Integer)return
Integeris
subtype
Random_Typeis
Integerrange
MinValue .. MaxValue;package
Random_Packis
new
Ada.Numerics.Discrete_Random (Random_Type); G : Random_Pack.Generator;begin
Random_Pack.Reset (G);return
Random_Pack.Random (G);end
Generate_Number;
Number_1 : Integer := Generate_Number (10);
Number_2 : Integer := Generate_Number (6, 10);
Function overloading in Ada
Ada supports all six signature options but if you use the arguments' name as option you will always have to name the parameter when calling the function. i.e.:
Number_2 : Integer := Generate_Number (MinValue => 6, MaxValue => 10);
Note that you cannot overload a generic procedure or generic function within the same package. The following example will fail to compile:
package
myPackagegeneric
type
Value_Typeis
(<>); -- The first declaration of a generic subprogram -- with the name "Generic_Subprogram"procedure
Generic_Subprogram (Value :in
out
Value_Type); ...generic
type
Value_Typeis
(<>); -- This subprogram has the same name, but no -- input or output parameters. A non-generic -- procedure would be overloaded here. -- Since this procedure is generic, overloading -- is not allowed and this package will not compile.procedure
Generic_Subprogram; ...generic
type
Value_Typeis
(<>); -- The same situation. -- Even though this is a function and not -- a procedure, generic overloading of -- the name "Generic_Subprogram" is not allowed.function
Generic_Subprogram (Value : Value_Type)return
Value_Type;end
myPackage;
See also
Wikibook
Ada 95 Reference Manual
Ada 2005 Reference Manual
This article is issued from
Wikibooks.
The text is licensed under Creative
Commons - Attribution - Sharealike.
Additional terms may apply for the media files.